Laravel Notificationでメールの送信先を変更する
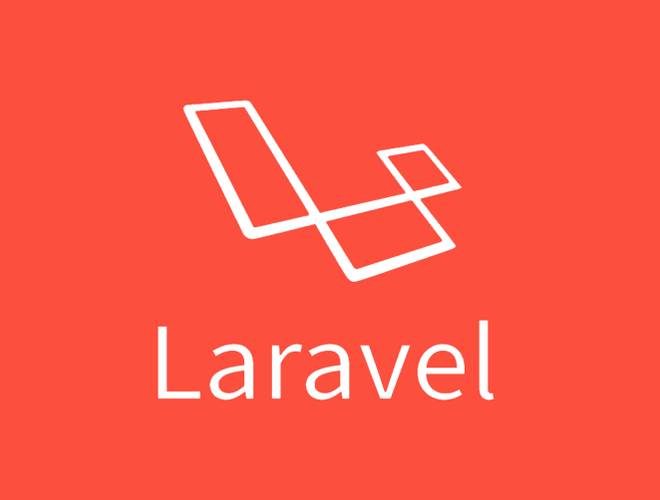
Laravel Notificationとは
ユーザーへメールなどで通知を行うためのLaravelの機能です。
e.g. 送信例
$user->notify(new \App\Notifications\Reserved($reservation));
通知クラスはmake:notification
Artisanコマンドを実行することで作成できます。
$ php artisan make:notification Reserved
アカウントごとのメールの送信先を変更する
メールの送信先はデフォルトではemail
プロパティに設定されているメールアドレスとなっていますが、routeNotificationForMail
メゾットをオーバーライドすることで変更できます。
e.g. 複数のメールアドレスに送信する例
<?php
namespace App;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use Notifiable;
//...
public function routeNotificationForMail(): array
{
return $this->emailsToArray();
}
public function emailsToArray(): array
{
$emails = [];
if ($this->email) {
$emails[] = $this->email;
}
if ($this->email2) {
$emails[] = $this->email2;
}
return $emails;
}
}
CC・BCCを設定する
MailMessage
クラスはCC, BCCを設定することができます。
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Notifications\Messages\MailMessage;
use Illuminate\Notifications\Notification;
class Reserved extends Notification
{
use Queueable;
//...
/**
* Get the mail representation of the notification.
*/
public function toMail($notifiable): MailMessage
{
return (new MailMessage)
->cc([ 'cc@example.com' ])
->bcc([ 'bcc@example.com' ])
->subject('Notification Subject')
->line('...');
}
}
通知内容ごとに送信先を変更する
MailMessage
クラスを使用した場合、送信先を変更することはできません。送信先を変更する場合には、MailMessage
クラスではなく、Mailable
クラスを用いて送信する必要があります。
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class MailMessage extends Mailable
{
use Queueable, SerializesModels;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct()
{
//
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this;
}
}
<?php
namespace App\Notifications;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use App\Mail\MailMessage as MailMessageMailable;
use Illuminate\Notifications\Notification;
class Reserved extends Notification
{
use Queueable;
//...
/**
* Get the mail representation of the notification.
*/
public function toMail($notifiable): MailMessage
{
return (new MailMessageMailable)
->to($notifiable->email)
->subject('Notification Subject')
->markdown('mail.reserved');
}
}