Laravelにユーザー作成コマンドを追加する
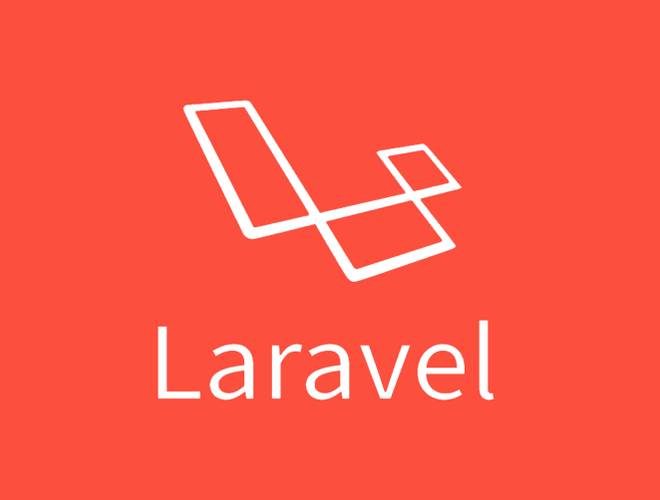
本日は、初期アカウントなどを作成するためのLaravelのArtisanコマンドの作成方法を紹介致します。
ユーザー作成用のArtisanコマンドがあると環境構築時にDBを直接触らずに済むので非常に便利です。
tinkerでもDBの操作はできますが、よく使用するものはArtisanコマンド化した方がラクかと思います。
実行環境
- PHP ^8.0 | ^8.1
- Laravel 9
注意事項
紹介するコードはLaravelのデフォルトのUser
モデル及びusers
テーブルを使用していることを前提としています。
Artisanコマンド作成
app/Console/Commands/CreateUserCommand.php
namespace App\Console\Commands;
use App\Models\User;
use Illuminate\Console\Command;
use Illuminate\Support\Facades\Hash;
use Illuminate\Support\Facades\Validator;
use Symfony\Component\Console\Exception\RuntimeException;
class CreateUserCommand extends Command
{
protected $signature = 'user:create {--u|username= : User name of the newly created user.} {--e|email= : E-mail of the newly created user.} {--p|password= : Password of the newly created user.}';
protected $description = 'Create a new user';
/**
* @var array{ email: string, password: string, name: string }
*/
protected array $attributes = [
'email' => 'info@example.com',
];
protected string $defaultPassword = 'password';
public function handle(): int
{
try {
$this->promptEmail();
$this->promptUsername();
$this->promptPassword();
$user = $this->createUser();
$this->info('User created successfully.');
} catch (RuntimeException $e) {
$this->error($e->getMessage());
return 1;
}
return 0;
}
protected function promptEmail(): static
{
$email = $this->option('email');
if ($email === null) {
$email = $this->ask('What is an E-mail?', $this->attributes['email']);
}
$validator = Validator::make([
'email' => $email,
], [
'email' => ['required', 'email', 'unique:users,email'],
]);
if ($validator->fails()) {
throw new RuntimeException($validator->errors()->first());
}
$this->attributes['email'] = $email;
return $this;
}
protected function promptUsername(): static
{
$name = $this->option('username');
if ($name === null) {
$name = $this->ask('What is a name?', $this->attributes['name'] ?? $this->attributes['email']);
}
$this->attributes['name'] = $name;
return $this;
}
protected function promptPassword(): static
{
$password = $this->option('password');
if ($password === null) {
$password = $this->secret(sprintf('What is a password? [%s]', $this->defaultPassword))
?? $this->defaultPassword;
}
$this->attributes['password'] = Hash::make($password);
return $this;
}
protected function createUser(): User
{
/** @var User $user */
$user = User::query()->create($this->attributes);
return $user;
}
}
app/Console/Kernel.phpに登録したコマンドを登録。
namespace App\Console;
use Illuminate\Console\Scheduling\Schedule;
use Illuminate\Foundation\Console\Kernel as ConsoleKernel;
class Kernel extends ConsoleKernel
{
protected $commands = [
\App\Console\Commands\CreateUserCommand::class,
];
// ...
}
コマンド確認
listコマンドで登録したartisanコマンドが登録されているか確認することができます。
$ php artisan list
...
user
user:create Create a new user
コマンド実行
対話型でユーザー登録。
$ php artisan user:create
What is an E-mail? [info@example.com]:
> admin@example.com
ユーザー情報を引数で指定することも可能です。
$ php artisan user:create -u admin -e admin@example.com
おわりに
READMEにアカウント作成コマンドが記載されているとローカル環境の構築がスムーズにできるのでおすすめです。