LaravelでSEO対策に必要なメタタグを自動生成する
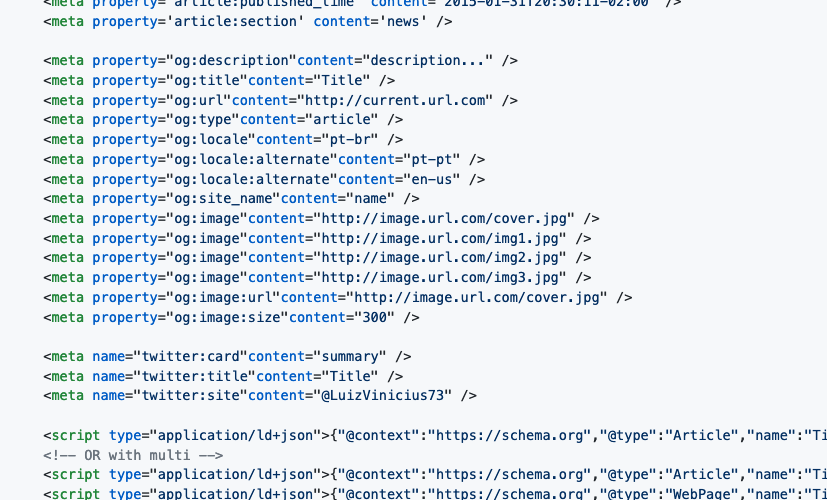
本日は、SEO対策に必要なメタタグを自動生成するLaravelのHelper関数を提供してくれるライブラリを紹介します。
目次
artesaos/seotools
artesaos/seotoolsは、SEO対策に必要なメタタグとTwitter Cards, Open GraphそしてJSON Linked Dataを自動生成してくれるLumenとLaravelに対応したSEO対策用ライブラリです。
インストール
composerでインストール後、config/app.php
にSEOToolsのproviders
とaliases
を追加します。
$ composer require artesaos/seotools
aliases
は、各種それぞれを登録するか、それらをひとまとめにしたSEO
から選択する事ができます。
// config/app.php
return [
// ...
'providers' => [
Artesaos\SEOTools\Providers\SEOToolsServiceProvider::class,
// ...
],
// ...
'aliases' => [
'SEOMeta' => Artesaos\SEOTools\Facades\SEOMeta::class,
'OpenGraph' => Artesaos\SEOTools\Facades\OpenGraph::class,
'Twitter' => Artesaos\SEOTools\Facades\TwitterCard::class,
'JsonLd' => Artesaos\SEOTools\Facades\JsonLd::class,
'JsonLdMulti' => Artesaos\SEOTools\Facades\JsonLdMulti::class,
// or
'SEO' => Artesaos\SEOTools\Facades\SEOTools::class,
// ...
],
// ...
];
デフォルト設定
デフォルトの設定は、config/seotools.php
で行います。
下記のコマンドでconfig/seotools.php
を生成してください。
$ php artisan vendor:publish --provider="Artesaos\SEOTools\Providers\SEOToolsServiceProvider"
各種設定
// config/seotools.php
return [
'meta' => [
/*
* The default configurations to be used by the meta generator.
*/
'defaults' => [
'title' => "It's Over 9000!", // set false to total remove
'titleBefore' => false, // Put defaults.title before page title, like 'It's Over 9000! - Dashboard'
'description' => 'For those who helped create the Genki Dama', // set false to total remove
'separator' => ' - ',
'keywords' => [],
'canonical' => false, // Set null for using Url::current(), set false to total remove
'robots' => false, // Set to 'all', 'none' or any combination of index/noindex and follow/nofollow
],
/*
* Webmaster tags are always added.
*/
'webmaster_tags' => [
'google' => null,
'bing' => null,
'alexa' => null,
'pinterest' => null,
'yandex' => null,
'norton' => null,
],
'add_notranslate_class' => false,
],
'opengraph' => [
/*
* The default configurations to be used by the opengraph generator.
*/
'defaults' => [
'title' => 'Over 9000 Thousand!', // set false to total remove
'description' => 'For those who helped create the Genki Dama', // set false to total remove
'url' => false, // Set null for using Url::current(), set false to total remove
'type' => false,
'site_name' => false,
'images' => [],
],
],
'twitter' => [
/*
* The default values to be used by the twitter cards generator.
*/
'defaults' => [
//'card' => 'summary',
//'site' => '@LuizVinicius73',
],
],
'json-ld' => [
/*
* The default configurations to be used by the json-ld generator.
*/
'defaults' => [
'title' => 'Over 9000 Thousand!', // set false to total remove
'description' => 'For those who helped create the Genki Dama', // set false to total remove
'url' => false, // Set null for using Url::current(), set false to total remove
'type' => 'WebPage',
'images' => [],
],
],
];
Usage
コントローラー
各ページごとにコントローラーでページごとの設定を行います。
<?php
namespace App\Http\Controllers;
use Artesaos\SEOTools\Facades\SEOMeta;
use Artesaos\SEOTools\Facades\OpenGraph;
use Artesaos\SEOTools\Facades\TwitterCard;
use Artesaos\SEOTools\Facades\JsonLd;
// OR with multi
use Artesaos\SEOTools\Facades\JsonLdMulti;
// OR
use Artesaos\SEOTools\Facades\SEOTools;
class CommomController extends Controller
{
public function index()
{
SEOMeta::setTitle('Home');
SEOMeta::setDescription('This is my page description');
SEOMeta::setCanonical('https://codecasts.com.br/lesson');
OpenGraph::setDescription('This is my page description');
OpenGraph::setTitle('Home');
OpenGraph::setUrl('http://current.url.com');
OpenGraph::addProperty('type', 'articles');
TwitterCard::setTitle('Homepage');
TwitterCard::setSite('@LuizVinicius73');
JsonLd::setTitle('Homepage');
JsonLd::setDescription('This is my page description');
JsonLd::addImage('https://codecasts.com.br/img/logo.jpg');
// OR
SEOTools::setTitle('Home');
SEOTools::setDescription('This is my page description');
SEOTools::opengraph()->setUrl('http://current.url.com');
SEOTools::setCanonical('https://codecasts.com.br/lesson');
SEOTools::opengraph()->addProperty('type', 'articles');
SEOTools::twitter()->setSite('@LuizVinicius73');
SEOTools::jsonLd()->addImage('https://codecasts.com.br/img/logo.jpg');
$posts = Post::all();
return view('myindex', compact('posts'));
}
public function show($id)
{
$post = Post::find($id);
SEOMeta::setTitle($post->title);
SEOMeta::setDescription($post->resume);
SEOMeta::addMeta('article:published_time', $post->published_date->toW3CString(), 'property');
SEOMeta::addMeta('article:section', $post->category, 'property');
SEOMeta::addKeyword(['key1', 'key2', 'key3']);
OpenGraph::setDescription($post->resume);
OpenGraph::setTitle($post->title);
OpenGraph::setUrl('http://current.url.com');
OpenGraph::addProperty('type', 'article');
OpenGraph::addProperty('locale', 'pt-br');
OpenGraph::addProperty('locale:alternate', ['pt-pt', 'en-us']);
OpenGraph::addImage($post->cover->url);
OpenGraph::addImage($post->images->list('url'));
OpenGraph::addImage(['url' => 'http://image.url.com/cover.jpg', 'size' => 300]);
OpenGraph::addImage('http://image.url.com/cover.jpg', ['height' => 300, 'width' => 300]);
JsonLd::setTitle($post->title);
JsonLd::setDescription($post->resume);
JsonLd::setType('Article');
JsonLd::addImage($post->images->list('url'));
// OR with multi
JsonLdMulti::setTitle($post->title);
JsonLdMulti::setDescription($post->resume);
JsonLdMulti::setType('Article');
JsonLdMulti::addImage($post->images->list('url'));
if(! JsonLdMulti::isEmpty()) {
JsonLdMulti::newJsonLd();
JsonLdMulti::setType('WebPage');
JsonLdMulti::setTitle('Page Article - '.$post->title);
}
// Namespace URI: http://ogp.me/ns/article#
// article
OpenGraph::setTitle('Article')
->setDescription('Some Article')
->setType('article')
->setArticle([
'published_time' => 'datetime',
'modified_time' => 'datetime',
'expiration_time' => 'datetime',
'author' => 'profile / array',
'section' => 'string',
'tag' => 'string / array'
]);
// Namespace URI: http://ogp.me/ns/book#
// book
OpenGraph::setTitle('Book')
->setDescription('Some Book')
->setType('book')
->setBook([
'author' => 'profile / array',
'isbn' => 'string',
'release_date' => 'datetime',
'tag' => 'string / array'
]);
// Namespace URI: http://ogp.me/ns/profile#
// profile
OpenGraph::setTitle('Profile')
->setDescription('Some Person')
->setType('profile')
->setProfile([
'first_name' => 'string',
'last_name' => 'string',
'username' => 'string',
'gender' => 'enum(male, female)'
]);
// Namespace URI: http://ogp.me/ns/music#
// music.song
OpenGraph::setType('music.song')
->setMusicSong([
'duration' => 'integer',
'album' => 'array',
'album:disc' => 'integer',
'album:track' => 'integer',
'musician' => 'array'
]);
// music.album
OpenGraph::setType('music.album')
->setMusicAlbum([
'song' => 'music.song',
'song:disc' => 'integer',
'song:track' => 'integer',
'musician' => 'profile',
'release_date' => 'datetime'
]);
//music.playlist
OpenGraph::setType('music.playlist')
->setMusicPlaylist([
'song' => 'music.song',
'song:disc' => 'integer',
'song:track' => 'integer',
'creator' => 'profile'
]);
// music.radio_station
OpenGraph::setType('music.radio_station')
->setMusicRadioStation([
'creator' => 'profile'
]);
// Namespace URI: http://ogp.me/ns/video#
// video.movie
OpenGraph::setType('video.movie')
->setVideoMovie([
'actor' => 'profile / array',
'actor:role' => 'string',
'director' => 'profile /array',
'writer' => 'profile / array',
'duration' => 'integer',
'release_date' => 'datetime',
'tag' => 'string / array'
]);
// video.episode
OpenGraph::setType('video.episode')
->setVideoEpisode([
'actor' => 'profile / array',
'actor:role' => 'string',
'director' => 'profile /array',
'writer' => 'profile / array',
'duration' => 'integer',
'release_date' => 'datetime',
'tag' => 'string / array',
'series' => 'video.tv_show'
]);
// video.tv_show
OpenGraph::setType('video.tv_show')
->setVideoTVShow([
'actor' => 'profile / array',
'actor:role' => 'string',
'director' => 'profile /array',
'writer' => 'profile / array',
'duration' => 'integer',
'release_date' => 'datetime',
'tag' => 'string / array'
]);
// video.other
OpenGraph::setType('video.other')
->setVideoOther([
'actor' => 'profile / array',
'actor:role' => 'string',
'director' => 'profile /array',
'writer' => 'profile / array',
'duration' => 'integer',
'release_date' => 'datetime',
'tag' => 'string / array'
]);
// og:video
OpenGraph::addVideo('http://example.com/movie.swf', [
'secure_url' => 'https://example.com/movie.swf',
'type' => 'application/x-shockwave-flash',
'width' => 400,
'height' => 300
]);
// og:audio
OpenGraph::addAudio('http://example.com/sound.mp3', [
'secure_url' => 'https://secure.example.com/sound.mp3',
'type' => 'audio/mpeg'
]);
// og:place
OpenGraph::setTitle('Place')
->setDescription('Some Place')
->setType('place')
->setPlace([
'location:latitude' => 'float',
'location:longitude' => 'float',
]);
return view('myshow', compact('post'));
}
}
SEOToolsTrait
SEOToolsTrait
を利用した場合は、下記のように書けます。
<?php
namespace App\Http\Controllers;
use Artesaos\SEOTools\Traits\SEOTools as SEOToolsTrait;
class CommomController extends Controller
{
use SEOToolsTrait;
public function index()
{
$this->seo()->setTitle('Home');
$this->seo()->setDescription('This is my page description');
$this->seo()->opengraph()->setUrl('http://current.url.com');
$this->seo()->opengraph()->addProperty('type', 'articles');
$this->seo()->twitter()->setSite('@LuizVinicius73');
$this->seo()->jsonLd()->setType('Article');
$posts = Post::all();
return view('myindex', compact('posts'));
}
}
View
メタタグを出力するためにBladeに下記を追加します。
<html>
<head>
{!! SEOMeta::generate() !!}
{!! OpenGraph::generate() !!}
{!! Twitter::generate() !!}
{!! JsonLd::generate() !!}
<!-- OR with multi -->
{!! JsonLdMulti::generate() !!}
<!-- OR -->
{!! SEO::generate() !!}
<!-- MINIFIED -->
{!! SEO::generate(true) !!}
</head>
<body>
</body>
</html>
出力結果
全て設定した場合、以下のようなコードが出力されるようになります。
<html>
<head>
<title>Title - Over 9000 Thousand!</title>
<meta name='description' itemprop='description' content='description...' />
<meta name='keywords' content='key1, key2, key3' />
<meta property='article:published_time' content='2015-01-31T20:30:11-02:00' />
<meta property='article:section' content='news' />
<meta property="og:description"content="description..." />
<meta property="og:title"content="Title" />
<meta property="og:url"content="http://current.url.com" />
<meta property="og:type"content="article" />
<meta property="og:locale"content="pt-br" />
<meta property="og:locale:alternate"content="pt-pt" />
<meta property="og:locale:alternate"content="en-us" />
<meta property="og:site_name"content="name" />
<meta property="og:image"content="http://image.url.com/cover.jpg" />
<meta property="og:image"content="http://image.url.com/img1.jpg" />
<meta property="og:image"content="http://image.url.com/img2.jpg" />
<meta property="og:image"content="http://image.url.com/img3.jpg" />
<meta property="og:image:url"content="http://image.url.com/cover.jpg" />
<meta property="og:image:size"content="300" />
<meta name="twitter:card"content="summary" />
<meta name="twitter:title"content="Title" />
<meta name="twitter:site"content="@LuizVinicius73" />
<script type="application/ld+json">{"@context":"https://schema.org","@type":"Article","name":"Title - Over 9000 Thousand!"}</script>
<!-- OR with multi -->
<script type="application/ld+json">{"@context":"https://schema.org","@type":"Article","name":"Title - Over 9000 Thousand!"}</script>
<script type="application/ld+json">{"@context":"https://schema.org","@type":"WebPage","name":"Title - Over 9000 Thousand!"}</script>
</head>
<body>
</body>
</html>